The Chat Application Second Upgrade
Ovr two weeks ago, I upgraded my chat application to have a user interface and create a notification everytime a new message arrives. Today, I am happy to announce that it got another upgrade. As a summary, changes implemented are the following:
- It handles multi clients similar to a chatroom. The previous version only allows 1 client at a time.
- The gui is now using Multiple Document Interface which allow users to chat with their friends on a separate subwindow or mdi child window with just a click, it is also the same with a room chat
- The friends list is now being retrieved from the back-end Mysql database.
- Users can broadcast the message to the whole group or sends the message to one member only
- Users have to enter their alias before connecting
- The server no longer participates in the chat, it now functions as a message broadcaster
- Users can connect and disconnect anytime.
- Users using different computers but connected on the same network may now communicate using this chat program.
- Added a Integrated Development Environment to facilitate bug tracing and simplify testing.
- Added a planner at the files list panel of the IDE to keep track of my daily activities and at a glance, I know where to start or what to do next.
The Multiple Document interface(MDI)
The MDI is a container that may contain several subwindows or mdi children. These subwindows may display a document or a data entry screens like creation of common business transactions but in this case, chat windows since I am developing primarily a chat application. Having said that, the creation of chat subwindows, communication may take place in several instances all at the same time involving several devices. The creation of subwindows in a chat application is a little bit more challenging because each subwindow will be created by the same code, which when several instances were opened at the same time may cause confusion, unlike in visual basic, creation of several instances of subwindows will automatically assign a unique index number for that instance making it easily distinguishable from the rest of all other instances, in PyQt6 it does not do that, but it can be easily achieved by isolating the definition of the contents of the subwindow making it(MDI container) aware of the unique instances of each individual subwindows.
The Communication Protocol
With the use of MDI, users can now open unlimited subwindows at the same time to communicate with their friends and participate in a discussion in their communities. Developing an application that involves communication between several devices, having too many instances all at the same time, and making sure that the message is delivered to the intended receiver, a communication protocol must put in place and it can not be avoided no matter how I make the program as simple as possible. In this application, I have implemented a four layer model on top of the existing protocol used by sockets. The first layer contains the type of intended recipient(It is either a friend or a room or a public chatroom), the second layer is the sender, the third layer is the recipient(it could be the friend, room or the public chatroom) and the fourth layer is the message. There is also a communication handshake wherein the server will send a keyword like in this case, I used 'USER' and the recipient will automatically send the entered alias. After the handshake, the server will broadcast that the user has joined the chat or is online. The message should contain a keyword 'JOIN'. There is also another keyword I used to notify the community that the user has left, and the keyword is 'ALERT'. This might change when the encryption feature is implemented. I have prepared a complete list of protocol or agreed communication format(see the 'protocol' on the left side panel if you are using the builtin IDE or the protocol.txt file at the root directory of this project) for details and guide how the design was implemented.
The Integrated Development Environment(IDE)
I am encountering a lot of bugs and having difficulty in testing(I have to create at least 3 instances of the chat application and another instance of the server application and I have to arrange/resize all these windows to fit on an 11.3-inch screen), it is such a tedious and repetitive task that makes the development of this chat application too complex and boring. To get rid of this issue, I have decided that I have to copy the IDE I have implemented on my PyQt6 Desktop Application Template. The original IDE consist of 2 buttons(Syntax Checker and Recompile), a text editor that displays the code and where the code is edited at the same time and at the bottom is the error pane. This IDE is already functioning well but I have further enhanced it to include line numbers at the code editor section which is quite difficult to implement but after several hours of research, I have managed to make the changes and improvements work. Another significant improvements on this new IDE are the capability of this IDE to edit text files(usually documentation files but planning to make more improvements in the future to include jinja2 templating to enable this IDE to be capable of producing documents that include images, embed videos and better graphical user interface. Note that I have edited this text document on this new IDE), I also added text-to-speech to help in proofreading the document, a separate list widget on the left panel that displays all the files used in this project which when clicked should display the code for editing and on the right most panel are list of variables that I want to monitor during debugging and below it is the document version management system(This is different from the planned version management mention on the next paragraph because it will just be for the numerous documentation files I am planning to maintain within this IDE) but this is still not working on this upgrade. I have observed that with more text documents being added as time goes by, this IDE is slowly evolving to include a Content management System which is a great idea to include this module into the IDE. Note that this IDE is created specifically for this project which means all files are hard coded. I may create a dedicated project just for this IDE which I think will increase productivity of many if not all Python Software Developers like me.
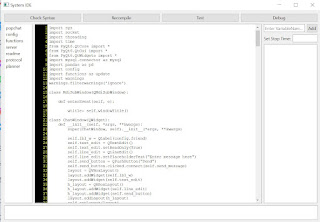
Features not implemented but planned to include in the next upgrade
- Messages should have three statuses(sent, delivered and read)
- Each message should have timestamp
- Unread messages should not be lost when another message arrives
- Messages should be saved on the MySql Database at the server so that when users login to a different terminal, he can still read the messages
- Messages should be saved to a local Sqlite3 database so that network will be minimized.
- A fifth layer in the communication protocol must be added and it should contain the encrypted password of the user
- A sixth layer in the communication protocol must be added and it should contain a code as key that to the server assigned challenge to the user and the terminal that user is using(still in draft therefore subject to change).
Planned Features
With these changes, the chat project is beginning to be a full chat application but I still need to add more features though like the ability of the server to moderate the chatroom, ban or kick out users that frequently sends spam messages, add encryption features so that messages will not be intercepted by hackers as seen on my previous post(A simulation of a Cyber Attack), the freinds and rooms and other data of the user should come from the server upon getting authenticated and will send to user then the client app will save the friends list and rooms list of the user to a local sqlite3 database(this will only happen when the user logs in from another terminal), add screen sharing and call conferencing, integrate an sms/email engine features, and so on, not to mention the features I have mentioned on my previous post which includes adding a user timeline, integrate that timeline to the user's facebook, twitter and linkedin timelines, add user management system(I gave an overview on this system on my previous post and has published a working prototype on my PyQt6 Desktop Application Template series), a project management system (this is composed of several modules like organizing a team, schedule a meeting, create a project repository, version management system, and reporting), add user dashboards(this should give the user a glimpse of what's happening in the community he joined, analyze his clout in the social media space by accessing his twitter, facebook and linkedin data and activities logs) and user appointment calendar. Other features I have thought to include are the following: there will be a community chatrooms organized according to certain topics and interests which is a good way to interact with strangers but you both share common interest like a discussion about astronomy, science, current events, etc. There might also be a market place where a user can create his own room exclusive for the merchandise he is selling. The seller can accept payment, issue invoice, official receipts, etc which is very much like a typical online stores like Lazada, Shopee and others. Add capability of a user to broadcast his messages across all rooms/chat windows depending on a user's previledges, this feature may also be used for important announcements. And last feature I have in mind is to enhance further the IDE with modules like interactive debugging, code inspector, code syntax hilighter, should have integration with github for version management and automation of uploading to my online github repository and so much more.
The File Structure and System Architecture
I know that the developers community look for this particular section in every article dealing with this kind of topics. I will just give an overview of the File Structure and System Architecture in this section. They look for this information because they consider this as the real heart and soul of the project. For me, I look for this section particularly because it determines the real value of the project. Just like in my on going Python-Flask User Management System, I will be adopting some of the file names, variable decalaration, updating of these global variables and the model-view-controller design pattern or also known infamously as clean architecture.
I am getting excited about the progress of this tiny project which slowly becoming a huge system that everyone can benefit from with its numerous features as envisioned by me.
If you made it this far, you have the previlidge to download the source code at my github page.
Popchat